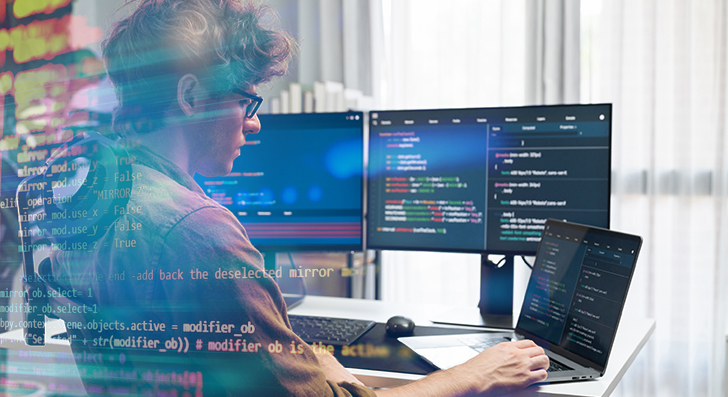
Scalability suggests your application can deal with growth—a lot more customers, more details, plus more site visitors—with out breaking. To be a developer, making with scalability in mind saves time and stress later on. Right here’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Style for Scalability from the Start
Scalability just isn't one thing you bolt on afterwards—it should be section of the approach from the beginning. Lots of programs fail every time they expand speedy due to the fact the first design and style can’t tackle the extra load. For a developer, you'll want to Believe early regarding how your procedure will behave under pressure.
Commence by building your architecture for being adaptable. Steer clear of monolithic codebases the place almost everything is tightly related. Rather, use modular style and design or microservices. These styles break your app into scaled-down, independent elements. Each module or support can scale By itself without the need of affecting The entire technique.
Also, give thought to your database from day a single. Will it need to deal with 1,000,000 end users or simply just 100? Choose the correct sort—relational or NoSQL—based upon how your details will expand. Prepare for sharding, indexing, and backups early, Even when you don’t have to have them yet.
Another significant issue is to avoid hardcoding assumptions. Don’t create code that only operates below existing problems. Give thought to what would materialize When your consumer foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use style and design styles that guidance scaling, like concept queues or celebration-driven techniques. These support your app deal with much more requests devoid of finding overloaded.
Any time you Create with scalability in mind, you're not just preparing for fulfillment—you might be cutting down foreseeable future problems. A nicely-planned procedure is less complicated to keep up, adapt, and expand. It’s much better to arrange early than to rebuild afterwards.
Use the best Database
Selecting the correct databases is often a essential A part of building scalable purposes. Not all databases are created the identical, and using the Completely wrong you can sluggish you down or perhaps induce failures as your application grows.
Begin by being familiar with your facts. Is it really structured, like rows in the desk? If Sure, a relational databases like PostgreSQL or MySQL is an efficient fit. These are definitely sturdy with relationships, transactions, and consistency. In addition they assist scaling procedures like read replicas, indexing, and partitioning to manage much more website traffic and info.
In the event your info is a lot more versatile—like person activity logs, product or service catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured info and will scale horizontally much more quickly.
Also, think about your read through and compose styles. Are you currently undertaking lots of reads with fewer writes? Use caching and browse replicas. Are you presently handling a weighty generate load? Consider databases that could deal with substantial generate throughput, or even occasion-dependent details storage systems like Apache Kafka (for short term knowledge streams).
It’s also good to Imagine forward. You may not will need Highly developed scaling attributes now, but selecting a database that supports them signifies you gained’t will need to modify afterwards.
Use indexing to hurry up queries. Stay away from unneeded joins. Normalize or denormalize your facts based upon your obtain styles. And always keep track of database overall performance as you develop.
In brief, the appropriate databases will depend on your application’s framework, pace demands, And just how you assume it to increase. Just take time to choose properly—it’ll help save many issues later on.
Enhance Code and Queries
Quick code is key to scalability. As your application grows, each individual smaller hold off provides up. Improperly written code or unoptimized queries can decelerate general performance and overload your process. That’s why it’s essential to Create productive logic from the start.
Get started by producing clear, straightforward code. Steer clear of repeating logic and take away something unnecessary. Don’t pick the most intricate Answer if a straightforward just one operates. Keep your capabilities quick, focused, and straightforward to test. Use profiling resources to find bottlenecks—destinations in which your code requires far too prolonged to run or works by using a lot of memory.
Next, have a look at your database queries. These generally sluggish things down in excess of the code itself. Be certain Each and every question only asks for the data you truly require. Prevent Find *, which fetches almost everything, and instead find certain fields. Use indexes to read more hurry up lookups. And steer clear of executing too many joins, Specially throughout big tables.
When you notice precisely the same details getting requested over and over, use caching. Retail store the outcomes briefly working with tools like Redis or Memcached and that means you don’t really have to repeat costly operations.
Also, batch your databases functions after you can. Rather than updating a row one after the other, update them in groups. This cuts down on overhead and tends to make your app additional economical.
Remember to check with huge datasets. Code and queries that get the job done great with 100 records may well crash whenever they have to handle 1 million.
In brief, scalable apps are rapidly applications. Maintain your code restricted, your queries lean, and use caching when wanted. These ways help your software keep sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more consumers and even more targeted traffic. If anything goes as a result of a person server, it will quickly turn into a bottleneck. That’s where by load balancing and caching are available. These two applications assistance keep the application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. Instead of one server accomplishing many of the get the job done, the load balancer routes end users to distinctive servers based upon availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the Other people. Tools like Nginx, HAProxy, or cloud-centered remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing details briefly so it may be reused rapidly. When buyers ask for exactly the same information yet again—like a product page or maybe a profile—you don’t must fetch it from the databases when. You may serve it within the cache.
There's two frequent types of caching:
one. Server-side caching (like Redis or Memcached) merchants information in memory for rapid accessibility.
two. Client-facet caching (like browser caching or CDN caching) shops static data files close to the consumer.
Caching reduces databases load, enhances velocity, and helps make your application much more successful.
Use caching for things that don’t transform often. And constantly make sure your cache is up-to-date when details does modify.
To put it briefly, load balancing and caching are straightforward but highly effective equipment. Together, they help your application deal with far more users, remain rapidly, and Get better from difficulties. If you intend to mature, you will need both equally.
Use Cloud and Container Tools
To construct scalable apps, you require tools that let your app increase conveniently. That’s exactly where cloud platforms and containers are available in. They provide you overall flexibility, cut down setup time, and make scaling A lot smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and expert services as you'll need them. You don’t must get components or guess long run potential. When targeted visitors increases, you are able to include much more sources with only a few clicks or instantly making use of automobile-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also give products and services like managed databases, storage, load balancing, and stability applications. You may center on making your application as an alternative to controlling infrastructure.
Containers are Yet another crucial Instrument. A container packages your application and anything it should run—code, libraries, settings—into a person unit. This can make it uncomplicated to maneuver your app between environments, from a laptop computer for the cloud, with out surprises. Docker is the preferred Resource for this.
Whenever your app takes advantage of many containers, equipment like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single portion of one's application crashes, it restarts it routinely.
Containers also allow it to be straightforward to independent parts of your application into providers. You can update or scale sections independently, which can be perfect for performance and dependability.
In brief, working with cloud and container resources usually means it is possible to scale fast, deploy easily, and Get well quickly when troubles occur. In order for you your app to increase with out boundaries, start employing these applications early. They conserve time, cut down danger, and make it easier to stay focused on making, not fixing.
Check Every thing
In case you don’t monitor your application, you gained’t know when matters go Incorrect. Monitoring can help the thing is how your app is executing, place challenges early, and make much better choices as your application grows. It’s a vital part of creating scalable devices.
Get started by tracking fundamental metrics like CPU utilization, memory, disk House, and response time. These tell you how your servers and providers are undertaking. Applications like Prometheus, Grafana, Datadog, or New Relic can help you collect and visualize this info.
Don’t just keep an eye on your servers—watch your application far too. Regulate how much time it takes for users to load pages, how often errors occur, and in which they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s occurring within your code.
Put in place alerts for critical challenges. One example is, If the reaction time goes previously mentioned a limit or even a support goes down, you ought to get notified right away. This assists you repair challenges speedy, generally in advance of end users even observe.
Monitoring is also useful after you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you can roll it again just before it leads to real problems.
As your app grows, traffic and details enhance. Without having checking, you’ll overlook signs of hassle right up until it’s as well late. But with the ideal equipment in place, you keep in control.
To put it briefly, checking assists you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about comprehension your method and making certain it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for big firms. Even small applications need a powerful Basis. By developing diligently, optimizing properly, and utilizing the correct applications, you'll be able to Make apps that grow easily without the need of breaking under pressure. Start out little, Consider big, and Construct smart.